Fixing SyntaxError Unexpected Token 'export'
Having issues with the error SyntaxError Unexpected Token 'export'. This post list ways to get rid of that!
Jan 21, 2023 | Read time 10 minutesđ Table of contents
Introduction
A common issue that comes up when I was working with single page apps with frameworks like Vue or React is the error: SyntaxError Unexpected Token âexportâ
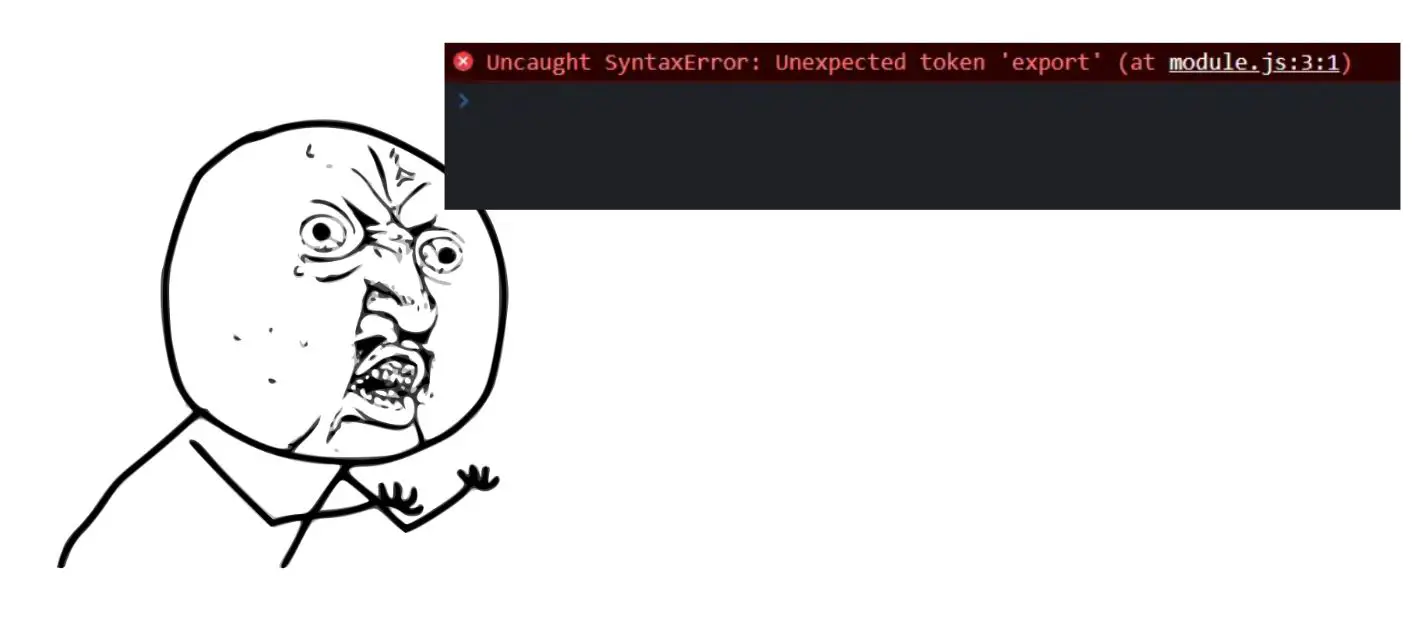
The export
keyword is from EcmaScript Module (ESM or âES6 Modulesâ) standard. The error SyntaxError Unexpected Token âexportâ comes up in your code because the environment that the code runs on does not support it. This can be front-end code (eg browser) or from a backend (Node JS)
Specifically, the âsyntaxerror unexpected token âexportâ" error is caused by any one of the following:
- In HTML, not setting the type attribute to equal module on the script tag -
<script type="module" src="index.js"></script>
- Not using the latest version of Node that supports ES6 standard
In this article, I will go over steps to address this error! Use the following checklist to fix the âsyntaxerror unexpected token âexportââ error in JavaScript:
-
Check browser support for ES6. The âexportâ keyword is part of the ECMAScript 6 (ES6) standard so make sure that browser will support this version!
-
Check the correct syntax - use the
type="module"
attribute in HTML -
Make sure you are not mixing CommonJs module with the
export
keyword -
For server-side JavaScript - verify the Node version supports ES6
What is the
export
keyword anyway?The
export
keyword allows you to make variables, functions, and objects available for use in other parts of your code by allowing them to be imported using the import keyword.
A simple way to use export is as follows - consider we have a Javascript file named moduleX.js:
export const serverUrl = 'example.com';
export const connectionString = 'blahblah';
Now, we have another file called index.js and we want to use the variables from the moduleX.js file, we just use it with the import
keyword.
So in our index.js file, we can have the following code:
import * as myModule from './myModule';
console.log(myModule.serverUrl);
// will print out example.com
1. Check browser support for ES6
ECMAScript 6 (ES6) is the sixth major release of the ECMAScript language specification. It was finalized in June 2015! It came with a bunch of improvements including the export
and import
keywords.
This forms the base for the modules functionality - providing a way to organize and reuse JavaScript code (previouly done through CommonJS)
However there are limitations on the support. Theres pretty much no support for ES6 functionality on IE11 or lower
2. Check the correct syntax - use the type="module"
attribute in HTML
Consider the following module.js javascript file - we will get the âUncaught SyntaxError: Unexpected token exportâ when we donât include the type=module
attribute:
//module.js:
function foo(){
return "foo";
}
var bar = "bar";
export { foo, bar };
Now if we include that in our HTML - the error will appear:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
</head>
<body>
<script src="module.js" /></script> <!-- â Avoid this - no type attribute -->
<script type="module" src="module.js"></script> <!-- âď¸ Added the type=module attribute -->
</body>
</html>
We can also use the import
keyword to use that module.js file (<script type="module" src="index.js"></script>
):
import { foo, bar } from "./module.js";
console.log( foo() );
console.log( bar );
Tip: Check for common JavaScript errors aswell!
I found this error can come up when our module JavaScript library got syntax errors. As an example below, we get a missing curly closing bracket:
if (true) {
/* â } missing here */
export function foo() {}
3. Make sure you are not mixing CommonJs module with the export
keyword
One common confusion when using the export
keyword is mix and matching it with CommonJS
const twitterClient = require("./twitterClient");
function search(tweet) {
return twitterClient.search(tweet);
};
export { search };
If we run the above, we can see:
export { search };
^^^^^^
SyntaxError: Unexpected token 'export'
Now if we are using CommonJS, we should not mix it with the export
keyword - we should use the export
object (confusing right? :P)
To fix the above code, we just replace the export
keyword with module.export
- so our final code looks like this:
const twitterClient = require("./twitterClient");
function search(tweet) {
return twitterClient.search(tweet);
};
module.export = { search };
What the heck is CommonJS?
Before ES6 and exports keyword, CommonJS is a project proposes a module system for JavaScript. This enables developers to organize their code into reusable modules and manage dependencies between them.
Its usually used in NodeJS projects with the module.exports
object to export things like functions, variables, etc. To use the exports we just use the require()
function.
An example of how to use it is below:
// math.js
module.exports = {
add: function(a, b) {
return a + b;
},
multiply: function(a, b) {
return a * b;
}
};
Then we have another file - index.js which uses the math.js file:
// Import the add function from the math module
var math = require('./math');
console.log(math.add(1, 2)); // Output: 3
4. For server-side JavaScript - verify the Node version supports ES6
Support for ES6 and the export keyword syntax (ESM) is only available in NodeJS v14.13.0.
Previously before NodeJS version 14.13.0, CommonJS modules are used - module.exports property syntax and require()
Some possible solutions:
- Upgrade to the latest version of Node which supports the export keyword syntax. If you are using NodeJS v14.13.0 or newer (which does support ESM) you can enable it by setting âtypeâ:âmoduleâ in your project package.json
- Refactor with CommonJS Module syntax (for older versions of NodeJS)
- Transpile the export keyword syntax to CommonJS using esbuild NPM package. This can be configured to transpile your ES6 javascript to a CommonJS pattern.
- Use babel to transpile your code to ES6.
Fixes for Syntax error unexpected token âexportâ for Express
A few common reasons why this error comes up in NodeJs or Express:
- You are using a old version of Node - make sure to use versions v14.13.0 or above so that ES6 features such as export/import are supported.
- You are mixing CommonJS (using require()) with the
export
keyword!
Tips for fixing React/Jest Syntax error Unexpected token âexportâ - transformIgnorePatterns
This error comes up in React projects when we run the Jest testing framework with React.
As an example below, previously I had a React app and decided to install DeckGl (https://github.com/visgl/deck.gl)
Then after install I got this lovely error when trying to run the test suite with Jest:
Test suite failed to run
/my_project/node_modules/deck.gl/src/react/index.js:21
export {default as DeckGL} from './deckgl';
^^^^^^
SyntaxError: Unexpected token export
at ScriptTransformer._transformAndBuildScript (node_modules/jest-runtime/build/script_transformer.js:318:17)
at Object.<anonymous> (node_modules/deck.gl/dist/react/deckgl.js:9:14)
at Object.<anonymous> (node_modules/deck.gl/dist/react/index.js:7:15)
To fix this we just need to update the jest.config.js file with the following configuration:
"transformIgnorePatterns": ["/node_modules/(?!deck\.gl)"]
By default Jest doesnât transform node_modules, because they should be valid JavaScript files. In our case DeckGL is written with ES6 therefore leading to syntax errors. So you have to tell this to Jest explicitly transform it:
// in the jest.config.js
{
"jest": {
"transformIgnorePatterns": [
"/node_modules/(?!deck\.gl)"
]
}
}
The above code just means that DeckGL will be transformed, even though they are node_modules.
Consideration for NextJS similar errors - next-transpile-modules
Consider using the next-transpile-modules
package when you see this error in your NextJS projects.
In the below scenario, I tried to add react-icons
to my NextJs project but when you build - it gives the error!.
The steps you can take to fix this are as follows:
- Open up your project directory and run the NPM install command:
npm i next-transpile-modules
- In your next.config.js file add the line
const transpiledModules = require('next-transpile-modules')(["react-icons"]);
Replace react-icons
with whatever package is failing for you.
The reason for this is the react-icons
package exports a function using ES6 import/export module. This fails in the browser since it does not know ES6 syntax and spits out Syntax error Unexpected token âexportâ
NextJs by default only transpiles code in the src folder and not in the node_modules folder. This is why we need the next-transpile-modules
package to transpile the dependency in node_modules from ES6!
Summary
Modules are a step up in managing large JavaScript codebases, however some of the errors that it spits out can be confusing.
This post I went over the annoying syntax unexpected token errors when working with modules in JavaScript. The reason for Uncaught Syntax error Unexpected token âexportâ error is mainly due to: - not using the the type=module
, using old versions of Node, running the JavaScript module code in a browser that does not support ES6, and mixing and matching with CommonJS require() and the new export/import keywords!