[Solved] NPM ERR Code err_socket_timeout
Steps to fix the dreaded NPM ERR Code err_socket_timeout
Feb 4, 2023 | Read time 11 minutes๐ Table of contents
Introduction
Recently, I was working on a fairly large front end application and encountered this error when I did a NPM install for all of the packages:
err_socket_timeout
This error is mainly due to a connection timeout with NPM install. The requested package took too long to respond and therefore cancels and you are left with the ERR_SOCKET_TIMEOUT.
This makes sense in my situation, since I was working on a laptop thats connected over 4G!
The error can look something like this in full:
npm ERR! code ERR_SOCKET_TIMEOUT
npm ERR! network Socket timeout
npm ERR! network This is a problem related to network connectivity.
npm ERR! network In most cases you are behind a proxy or have bad network settings.
npm ERR! network
npm ERR! network If you are behind a proxy, please make sure that the
npm ERR! network 'proxy' config is set properly. See: 'npm help config'
To fix this error ERR_SOCKET_TIMEOUT, we can try some of the following actions:
- Upgrade to the latest node and npm versions
- Check your proxy settings
- Check that you have internet connectivity
- Verify to use the
https
version of npm registry url - Update request timeout settings
npm config set fetch-timeout
- Use yarn to install packages instead of npm
- Do a clean install
1. Upgrade to the latest node and npm versions
Typically, whenever I see a npm or node issue, the first step to have a look at is checking your current npm and node versions and updating if required.
Firstly, open up the terminal and run the following commands to check npm and your node versions:
npm --version
node --version
If your NPM version is out of date, you can run the following command line to update the latest:
npm install npm@latest -g
The above will install the latest version of NPM globally.
2. Check your proxy settings
A common reason why this error comes up is that your proxy settings are not correct. This is usually the case when you have to run things behind a corporate proxy:
npm config set proxy http://yourproxyserver:8080
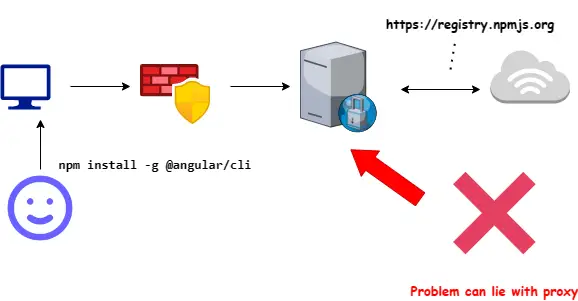
To verify that you have the right proxy settings we can do the following steps:
Remove current proxy settings
Firstly, use the commands to remove your current proxy settings (rm
)
npm config rm proxy
npm config rm https-proxy
We are removing both the proxy
config value and the https-proxy
values.
Set new proxy settings
The following commands will set your proxy settings. Open up the terminal and run:
npm config set proxy http://proxyurl:8080
You can replace the proxyurl
and port with your companyโs proxy url and port. Now if your proxy requires authentication, we can do the following formats:
npm config set proxy http://username:password@proxyurl:8080
npm config set https-proxy http://username:password@proxyurl:8080
Keep in mind that when you are using username and password, they need to be encoded. For example, if your password is: Welcome@12# then it will be like Welcome%4012%23.
Additionally, with your username, you may need to also include the domain name + username aswell.
For example, lets say we work at a company with domain BIGCORP
and your username is johnnyweekend
with password Welcome@12#, then your NPM proxy config might look something like this:
npm config set proxy http://bigcorp\\jonnyweekend:Welcome%4012%23@bigcorpproxy:8080
Tip: Check your corporate proxy settings and make sure that they are not blocking NPM registry
Check with your corporate network team that the proxy is not blocking the following URL: https://registry.npmjs.org
3. Check that you have internet connectivity
A more obvious reason why we are getting the ERR_SOCKET_TIMEOUT error is that our internet connection is out! Doh! This happened to me more times than not.
I was going through so many troubleshooting steps and just to realize that the internet connection doesnโt even work.
A quick tip is just to use the ping command:
Open up the terminal and run the following:
ping registry.npmjs.org
C:\Users\<user>>ping registry.npmjs.org
Pinging registry.npmjs.org [104.16.25.35] with 32 bytes of data:
Reply from 104.16.25.35: bytes=32 time=6ms TTL=60
Reply from 104.16.25.35: bytes=32 time=5ms TTL=60
Reply from 104.16.25.35: bytes=32 time=4ms TTL=60
Reply from 104.16.25.35: bytes=32 time=5ms TTL=60
Ping statistics for 104.16.25.35:
Packets: Sent = 4, Received = 4, Lost = 0 (0% loss),
Approximate round trip times in milli-seconds:
Minimum = 4ms, Maximum = 6ms, Average = 5ms
This we can confirm if our connection is down or NPM registry is down (registry.npmjs.org)
4. Verify to use the https
version of npm registry url
One issue that I see sometimes is that people somehow still hang onto the http
version of the NPM registry url
http://registry.npmjs.org
Going forward, NPM suggests that people should of moved over to the https
version of their url. We can configure it like so:
npm config set registry https://registry.npmjs.org/
If you are unsure of what registry url you are currently using, we can use the following command:
npm get registry
5. Update request timeout settings npm config set fetch-timeout
NPM comes with a bunch of settings that you can change to in regards to the max and min times for each fetch call:
We can configure the maximum time allowed for HTTP requests to get packages or the number of retries to allow.
Obviously we want to have a right balance - having too high values can hang your machine and too long could cause this error when your internet connection is not good:
fetch-retries = <number>
- the number of retries for HTTP requests. Default: 2fetch-retry-factor = <number>
- the retry factor. Default: 10fetch-retry-maxtimeout = <number>
- the max timeout for retries. Default: 60000 (1 minute)fetch-retry-mintimeout = <number>
- the minimum timeout for retries. Default: 10000 (10 seconds)fetch-timeout = <number>
- the max time for a HTTP request to complete. 300000 (5 minutes)
You can view your default values with the command:
npm config ls -l
As an example, the following commands can be used to update our max timeouts and retries to be higher than the defaults:
Open up your terminal and enter in the following settings:
npm config set fetch-retries 5
npm config set fetch-retry-factor 20
npm config set fetch-retry-mintimeout 20000
npm config set fetch-retry-maxtimeout 120000
npm config set fetch-timeout 600000
6. Use yarn to install packages instead of npm
More common than not, to fix NPM issues is to move away from NPM. We can try to use yarn to solve our problems here:
We can install yarn with the following NPM install command:
npm i -g yarn@latest
Then to install the packages and their dependencies, we can use one of the following:
yarn
yarn install
From experience, yarn works pretty well on slow connections. When there is a slow connection and it fails to download, it remembers the status would always try to reconnect and continue its progress from where it stopped.
7. Do a clean install - remove node_modules
and package-lock.json
If the above steps did not work for you we can try the nuke approach - removing the node_modules folder and package-lock.json file!
-
Firstly open up your terminal and go to the root of the project.
-
We need to delete the /node_modules with the following command (you might need to use
sudo
before each command if you are on a Linux distro):
rm -rf node_modules
- Delete package-lock.json file using the rm command:
rm -rf package-lock.json
- Install the dependencies using the following command:
npm install
Tip: Try clearing NPM cache
We can try running
npm cache clear --force
to clear the NPM cache. NPM keeps all of the packages and their dependencies in a local cache folder on your machine. This helps it speed things up the next time you get the same package.However, I have found it gets itself in a knot more often than not so I just clear it when you get random errors prop up.
Fix for React apps
Originally, I found this error ERR_SOCKET_TIMEOUT with my React app:
create-react-app jsx
Installing packages. This might take a couple of minutes.
Installing react, react-dom, and react-scripts with cra-template...
npm ERR! code ERR_SOCKET_TIMEOUT
npm ERR! errno ERR_SOCKET_TIMEOUT
npm ERR! request to https://registry.npmjs.org/babel-preset-react-app failed, reason: Socket timeout
npm ERR! A complete log of this run can be found in:
npm ERR! /home/xxxx/.npm/_logs/2020-12-23T00_02_40_003Z-debug.log
Aborting installation.
npm install --save --save-exact --loglevel error react react-dom react-scripts cra-template has failed.
Deleting generated file... package.json
Deleting jsx/ from /home/xxxx/Desktop/Modern_react_kurs
Done.
One way to fix this for all projects is to update the .npmrc file. This is just a configuration file that applies for npm.
Bumping up the timeout value can help in this case. As an example, open up the .npmrc file and update the
timeout=60000
Alternatively, you can use Yarn. If you are using yarn you can try:
yarn create react-app your-app-name
Summary
In this post, I went over the issue of getting ERR_SOCKET_TIMEOUT when running a NPM install on your project.
This error is usually caused by a network timeout issue or if not there are a few things we can try to fix this.
Firstly, make sure that our version of NPM is the latest version, if you are behind a corporate proxy, check the proxy settings, update the NPM timeout settings such as fetch-timeout
and fetch-retries
.
If the nothing else works, we can try to clear the node_modules and package-lock.json files and reinstall. Alternatively we can have a look at using Yarn as our package manager instead of NPM!