[Fix] CSS text overflow ellipsis not working
This post will go over how to fix your text-overflow:ellipsis not working issues
Dec 9, 2022 | Read time 9 minutes🔔 Table of contents
When creating web component that contains long text, we can use the text-overflow:ellipsis
CSS property to clip the content so that it does not
ruin our design.
For example, when you have a gallery grid with image titles, when we have a very long title it can stuff up the layout.
To get around this we can use the text-overflow:ellipsis
to add a ...
when the text is longer than the specified width of the grid.
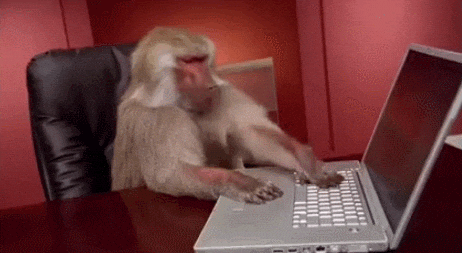
Reasons text-overflow:ellipsis
is not working in your code
- Check the containing element has a width value set. Only explicit units such as
px
,em
,rem
will work. Percentage units (%
) will not work here! - Will not work when the containing element does not have
overflow:hidden
ANDwhite-space:nowrap
set.
What is text-overflow:ellipsis used for?
Generally, when we use this technique to add a ellipis (
...
) to clip our long content. This can be useful for grid or card components, when the text is too long that could break your layout.More information here https://developer.mozilla.org/en-US/docs/Web/CSS/text-overflow
Steps to fix text-overflow:ellipsis
issues
- Review the containing element and add a
width
(ormax-width
)value that is not percentage (%) - Add
overflow:hidden
ANDwhite-space:nowrap
- Check the HTML tag default
display
property. Tags such as<a>
have a default ofdisplay:inline
. This does not support widths and therefore thetext-overflow:ellipsis
will not work!
Review the containing element and add a width
value that is not percentage (%)
Take the following example HTML with long text:
<a href="/somepage">Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut</a>
And we have the following CSS that is not working and we want to make the text cut off with an ellipsis (...
). We need to a widht
or max-width
value:
.a {
width: 100px; /* ✔️ Use pixel width instead of percentages */
overflow: hidden; /* ✔️ Required for ellipsis to appear */
text-align: center;
text-decoration: none;
text-overflow: ellipsis;
white-space: nowrap; /* ✔️ Required for ellipsis to appear */
display: inline-block; /* ✔️ display should have a value that supports width */
}
This will give the result that looks like the following:
💡 Tip: Hack to use % percentages
If you really want to use percentages instead of the explicit units such as pixels
px
for your widths, we can use thecalc()
method.For example, if you want 80% width, we can do
width:calc(80%)
. The calc() function ultimately converts the values to pixels which will work!
.a {
width: calc(80%); /* ✔️ Calc() will convert the percentage into pixels */
overflow: hidden;
text-overflow: ellipsis;
white-space: nowrap;
display: inline-block;
}
Adding overflow:hidden
AND white-space:nowrap
For text-overflow:ellipsis
to work correctly, we need to have the following properties set:
-
overflow:hidden
overrides the default behaviour when the content of an element overflows its width/ height. In this case, we want to hide/clip the content if it is larger than the container. This value will not show any scrollbars or give the user opportunity to scroll. -
white-space:nowrap
tells to the browser to collapse white space (change multiple white spaces in a piece of text into one space) and suppress line breaks.
Check the HTML tag default display
property
Since text-overflow:ellipsis
requires you to explictly set the width of the element, we have to review the display
property of the element.
Elements that have display:inline
as their default behaviour will not work. This is because display:inline
will not allow you to set the width of the element -
and this will be required by text-overflow:ellipsis
.
One way to get around this is to set the display to display:inline-block
or display:block
.
So when trying to add a ellipsis, make sure to review the following HTML tags that have display:inline as their default.
<a>
<abbr>
<acronym>
<audio> (if it has visible controls)
<b>
<bdi>
<bdo>
<big>
<br>
<button>
<canvas>
<cite>
<code>
<data>
<datalist>
<del>
<dfn>
<em>
<embed>
<i>
<iframe>
<img>
<input>
<ins>
<kbd>
<label>
<map>
<mark>
<meter>
<noscript>
<object>
<output>
<picture>
<progress>
<q>
<ruby>
<s>
<samp>
<script>
<select>
<slot>
<small>
<span>
<strong>
<sub>
<sup>
<svg>
<template>
<textarea>
<time>
<u>
<tt>
<var>
<video>
<wbr>
How to fix text-overflow:ellipsis
issues with flex layouts
When dealing with flex layouts, sometimes the text-overflow:ellipsis
does not work as we expect. When we have a long text and want to clip it will
ellipsis, the flex column appears to be wider than it should be!
Open the following codepen in another page to see the full effect:
As we can see in the above example, the first two examples do not behave as we expect it to. The first two examples, we can see that the clipped text expands the column width!
To fix this we add the min-width:0
property to the parent element that contains the text (or the outer most container that the width is overflowing)!
.action {
border: 1px dashed black
color: black
flex-shrink: 1
flex-grow: 1
max-width: 100%
padding: 1em
text-decoration: none;
min-width:0; /* ✔️ Add this to fix flex width issues */
}
💡 Tip: Modern approach for dynamic widths is to use grids
If your content is expected to be responsive and contains dynamics width, I would recommend to use grids!
.parent {
display: grid;
grid-template-columns: auto 1fr;
}
.long-text {
white-space: nowrap; /* ✔️ Required for ellipsis */
text-overflow: ellipsis; /* ✔️ Required for ellipsis */
overflow: hidden; /* ✔️ Required for ellipsis */
}
<div class="parent">
<div class="long-text">
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut
</div>
</div>
text-overflow:ellipsis
fix for multiple lines
If we want to have ellipsis on multiple lines, we cant use text-overflow:ellipsis
. This is because it was designed with single line texts in mind.
There is one way to do this for multi-line, but it will not be supported for non-webkit browsers (eg Firefox and IE).
We can use the -webkit-line-clamp
browser prefix:
💡 Note: As of Firefox version 68,
-webkit-line-clamp
is supported!https://developer.mozilla.org/en-US/docs/Mozilla/Firefox/Releases/68#css
.block-with-text {
overflow: hidden;
display: -webkit-box;
-webkit-line-clamp: 3;
-webkit-box-orient: vertical;
}
For Opera browsers, the equivalent fix to have ellipsis on multiline is text-overflow: -o-ellipsis-lastline
:
.last-line {
height: 3.6em; /* exactly three lines */
text-overflow: -o-ellipsis-lastline;
}
Bootstrap version for text-overflow:ellipsis
If you are working with Bootstrap for your web designs, the framework comes with a built in util class to truncate/ create ellipsis for long texts.
This is the text-truncate
class and simlar to our previous example, will require the element to have display of inline-block
or block
to work:
<!-- Block level -->
<div class="row">
<div class="col-2 text-truncate">
Praeterea iter est quasdam res quas ex communi.
</div>
</div>
<!-- Inline level -->
<span class="d-inline-block text-truncate" style="max-width: 150px;">
Praeterea iter est quasdam res quas ex communi.
</span>
Browser support
text-overflow:ellipsis
is generally available on the majority of modern browsers. There are some issues to consider when using this CSS property:
- Does not work in IE8 and IE9 on
<input type="text">
- Does not work on
select
tag for Chrome and IE. Will work only for Firefox. - For Samsung browsers, they will have issues with the
text-overflow:ellipsis
unlesstext-rendering
is not set toauto
.
Summary
In this post, we went over several reasons why using text-overflow:ellipsis
is not working for your code and several ways to address this.
Generally, when using text-overflow:ellipsis
, we need to specify the width
or max-width
and have the following properties set: overflow:hidden
and white-space: nowrap
.
If we want to have ellipsis with multilines, then we need to use vendor prefixes such as -webkit-line-clamp
for WebKit based browsers. For opera browsers,
we can use the equivalent of text-overflow: -o-ellipsis-lastline;