Solve proxy issues with - NPM reset proxy
Fix proxy issues with NPM reset proxy
Feb 23, 2023 | Read time 10 minutes🔔 Table of contents
Introduction
When I am starting working in a new place, I always get stumped by NPM an proxy settings.
Generally most corporate environments, the infrastructure/ networking admins want all software to go through their proxy (including NPM)
A common action is to reset the NPM proxy settings!
Usually, when we see something like the below error, we know that our proxy setting is not right and need to reset it!
In the below example, I am trying to do a npm install nodemon
but getting the error npm ERR! code ENOTFOUND
npm install nodemon
npm ERR! code ENOTFOUND
npm ERR! errno ENOTFOUND
npm ERR! network request to https://registry.npmjs.com/nodemon failed, reason: getaddrinfo ENOTFOUND 28
npm ERR! network This is a problem related to network connectivity.
npm ERR! network In most cases you are behind a proxy or have bad network settings.
npm ERR! network
npm ERR! network If you are behind a proxy, please make sure that the
npm ERR! network 'proxy' config is set properly. See: 'npm help config'`
If we look at a more verbose log file, it could look something like this:
216 error network tunneling socket could not be established, cause=connect ETIMEDOUT
216 error network This is most likely not a problem with npm itself
216 error network and is related to network connectivity.
216 error network In most cases you are behind a proxy or have bad network settings.
216 error network
216 error network If you are behind a proxy, please make sure that the
216 error network 'proxy' config is set properly. See: 'npm help config'
What is a proxy??
Proxies are usually used by corporates as middleman/ gateway for internet traffic going from your computer and the data from the external website back to your machine.
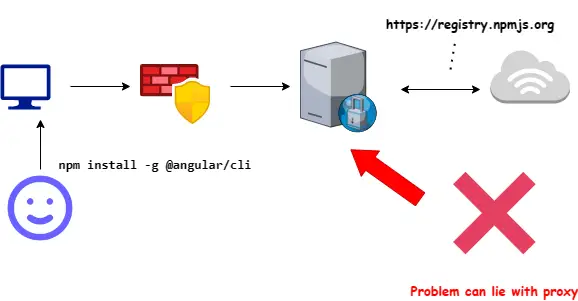
So why do we need this middleman - why cant I use it directly like I am doing it on my home laptop?
Well this is mostly to do with security and privacy. They want to filter out the traffic to make sure it is safe and keeping the internal networks away from any malware that could of been downloaded.
How do I reset my proxy settings for NPM 👈
To reset the proxy settings for NPM, you can use the following command in your terminal:
npm config rm proxy
npm config rm https-proxy
The above command will delete your proxy settings for HTTP and HTTPS proxy connections. As a result, NPM will not go through your proxy after the command executed successfully.
Now this could mean that you may not be able to access the internet or certain sites if accessing those sites was only through the proxy before.
Reset proxy HTTP_PROXY
and HTTPS_PROXY
environment variables
Proxy settings can also be done through environment variables.
If you previously set the proxy using an environment variable, you can unset it by using the following command (in Linux or OSX systems):
unset HTTP_PROXY
unset HTTPS_PROXY
If you are on a Windows system, you can do the following:
-
Open the command line (CMD)
-
Run:
set http_proxy=
set https_proxy=
Retrieving the NPM proxy settings
In cases where you do not need to reset the NPM proxy settings and just want to see your current settings, you can use the following get command:
npm config get proxy
The above command will return your HTTP proxy url. If there are no settings - ie you are not using a proxy, this will return null
As for the HTTPS proxy url we can use the below command instead:
npm config get https-proxy
If you are on windows and do not know your proxy url, then you can do:
reg query "HKEY_CURRENT_USER\Software\Microsoft\Windows\CurrentVersion\Internet Settings" | find /i "proxyserver"
This will return the current configured proxy value from your windows registry!
How to set NPM proxy
In case that the proxy setting we currently have is not working for NPM and you are getting errors like:
npm ERR! network If you are behind a proxy, please make sure that the
npm ERR! network 'proxy' config is set properly. See: 'npm help config'`
We can update our proxy settings with the set
value for NPM
npm config set proxy http://proxyurl:port_number
The above sets the proxy setting for HTTP. An example setting is:
npm config set proxy http://myproxy.com:8080
If your corporate environment uses a secure HTTPS proxy, we just need to update the command to use https_proxy
instead:
npm config set https-proxy http://proxyurl:port_number
With some proxies, it will require you to login with your username and password. If that is the case, we can add the username:password@
to your proxy url:
npm config set proxy http://username:password@proxyurl:port_number
npm config set https-proxy http://username:password@proxyurl:port_number
Keep in mind you have to have a valid user name and password that can authenticate with this proxy!
Additionally you may need to include the DOMAIN of your organisation as well:
npm config set proxy "http://domain\username:password@servername:port/"
Make sure you encode the url aswell. If your password contains special characters such as “,@,: and so on, replace them by their URL encoded values.
For example, the following special characters can be replaced as:
"
->%22
,@
->%40
,:
->%3A
.
Now if we want backslashes (\
) we can use %5C
.
Usernames and password in proxy environment variables
To set a proxy for NPM using an environment variable, you can use the HTTP_PROXY or HTTPS_PROXY variable, depending on whether you need to set the proxy for HTTP or HTTPS connections.
For example, to set a proxy for HTTP connections, you can use the following command in your terminal (on Linux environments):
export HTTP_PROXY=http://proxyurl:port_number
To set a proxy for HTTPS connections, use the following command instead:
export HTTPS_PROXY=http://proxyurl:port_number
Replace proxyurl
with the address of your proxy server and port_number with the port number used by the proxy server.
Setting HTTP_PROXY and HTTPS_PROXY for Windows
SET HTTP_PROXY=http://username:password@proxyurl:port
SET HTTPS_PROXY=http://username:password@proxyurl:port
If your proxy server requires authentication, you can add your username and password to the commands like this:
export HTTP_PROXY=http://username:password@proxyurl:port_number
export HTTPS_PROXY=http://username:password@proxyurl:port_number
Tip: Encode username and passwords
Make sure to replace username and password with your actual login credentials for the proxy server.
Keep in mind that when you are using username and password, they need to be encoded. For example, if your password is: Welcome@12# then it will be like Welcome%4012%23.
Additionally, with your username, you may need to also include the domain name + username aswell.
For example, lets say we work at a company with domain
BIGCORP
and your username isjohnnyweekend
with password Welcome@12#, then your NPM proxy config might look something like this:
npm config set proxy http://bigcorp\\jonnyweekend:Welcome%4012%23@bigcorpproxy:8080
Pass authentication to your proxy with Fiddler
If you are uncomfortable exposing your username and password like the above, we can use a middleman software like Fiddler to connect to your proxy.
So the above npm proxy settings can be updated to:
npm config set proxy http://localhost:8080
npm config set https-proxy http://localhost:8080
By installing Fiddler, we can run it on http://localhost:8080
and that it will point to our corporate proxy of http://proxyname:8080
To pass the credentials we just need to update Fiddler with the following setting (Rules -> Automattically Authenticate):
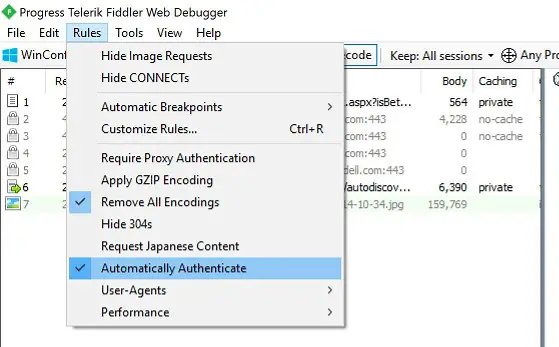
Tip: Proxy settings precedence with NPM
So which proxy setting will NPM use then? Theres so many places that it could appear, eg NPM config, environment variables, etc. The order that NPM uses proxy settings are as follows:
- Command-line parameters: This will override any other setting when using NPM on the command line:
For example:
npm install --proxy http://proxyurl:port_number
Environment variables: The
HTTP_PROXY
orHTTPS_PROXY
environment variables will be used NPM if they have been set.The
.npmrc
file: If there are proxy values set in the.npmrc
file, then NPM will use this as the third preference. NPMRC files can be global, user specific or project level
Summary
This post I went over how to reset proxy settings in NPM. We usually want to reset proxy settings if theres issues with the npm install
or to fix any existing proxy settings.
We can reset the proxy setting with npm config rm proxy
or npm config rm https-proxy
for HTTP and HTTPS proxies respectively.
Proxy settings can also appear in the HTTP_PROXY and HTTPS_PROXY environment variables and we can reset them with unset HTTP_PROXY
and unset HTTPS_PROXY
commands!