Fixing DataTables Pagination CSS not working correctly
Getting CSS to work on DataTables pagination could be tricky. We can go over a few steps to resolve this.
Jan 2, 2023 | Read time 7 minutes🔔 Table of contents
Introduction
This post we will over several reasons why your datatables pagination CSS is not loading correctly. You are just seeing something like the following image - just the numbers:
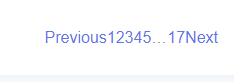
The main reason why datatables pagination CSS styles is not loading correctly is mainly due to the order that you load the external JavaScript! Since DataTables is a jQuery plugin we need to first load jquery and then the DataTables scripts!
DataTables quick overview
Simply put, DataTables is a JQuery plugin (https://datatables.net/) that is used widely to have interactive table data within your website or app. It comes with paging, serverside requests, searching and filtering out of the box. This helps reduce development time!
Reason 1 - Not loading jquery
Since DataTables is a jQuery plugin, we need to load jQuery before everything else. A common error that you can see is:
$ is undefined
in the console.
From the DataTables documentation, if we use the CDN option, it is suggested to load first the CSS, and then the DataTables external JavaScript library:
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.12.0/jquery.min.js">
</script> <!-- MAKE SURE THIS IS LOADED -->
<link
rel="stylesheet"
type="text/css"
href="https://cdn.datatables.net/1.13.1/css/jquery.dataTables.css">
<script
type="text/javascript"
charset="utf8"
src="https://cdn.datatables.net/1.13.1/js/jquery.dataTables.js">
</script>
Then we make sure to initialize DataTables AFTER the above has loaded:
<script>
$(document).ready(function () {
$('#example').DataTable();
});
</script>
Reason 2 - Not including the DataTables CSS file
A common reason why the CSS for DataTables pagination is not working correctly is that the CSS file was not included in your local bundle.
There are a few ways to install DataTables for your website. These include:
- Using a CDN
- Downloading it from https://datatables.net/download/ and use it locally
- Using it part of a build pipeline such as NPM or webpack
DataTables comes with two components - the JavaScript library and the CSS file.
For CDN installation option, we need to make sure we use the https://cdn.datatables.net/1.13.1/css/jquery.dataTables.css
:
<link rel="stylesheet" type="text/css" href="https://cdn.datatables.net/1.13.1/css/jquery.dataTables.css">
For local installs from the download page https://datatables.net/download/, make sure to check the datatables.min.css
<link rel="stylesheet" type="text/css" href="DataTables/datatables.min.css"/>
<script type="text/javascript" src="DataTables/datatables.min.js"></script>
As for using build tooling such as NPM, we need to have the following packages for styling:
npm install datatables.net # Core library
npm install datatables.net-dt # Styling
Reason 3 - Loading scripts in wrong order
Sometimes we can see that the CSS pagination is not loading correctly due to the order of how we load up the DataTable external scripts!
We need to make sure that we load jQuery first and then the DataTables CSS and finally followed by the DataTables JS!
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.12.0/jquery.min.js"></script>
<link rel="stylesheet" type="text/css" href="https://cdn.datatables.net/1.13.1/css/jquery.dataTables.css">
<script type="text/javascript" charset="utf8" src="https://cdn.datatables.net/1.13.1/js/jquery.dataTables.js"></script>
Now if you are using a build tool like NPM, you will need to make sure to also install jquery like so:
npm install --save jquery@1.12.3
npm install --save datatables.net-dt # This is the styling and datatables core js
And then references them like the following code:
var $ = require( 'jquery' );
var dt = require( 'datatables.net' )( window, $ );
Reason 4 - Not targeting the correct element
One reason why your pagination styles is not looking as intended is the targeting of the styles is not correct.
The pagination button CSS class is .paginate_button
. So for example, to add a circular pagination style, we can do the following:
.dataTables_wrapper .dataTables_paginate .paginate_button {
border-radius: 50% !important;
padding: 0.5em 0.9em !important;
}
DataTables pagination styles with bootstrap 4 issues
When using DataTables with Bootstrap 4, we need to make sure that we use the following includes:
<link rel="stylesheet" type="text/css" href="https://cdnjs.cloudflare.com/ajax/libs/twitter-bootstrap/4.6.0/css/bootstrap.min.css"/>
<link rel="stylesheet" type="text/css" href="https://cdn.datatables.net/v/bs4-4.6.0/jqc-1.12.4/dt-1.13.1/datatables.min.css"/>
<script type="text/javascript" src="https://cdn.datatables.net/v/bs4-4.6.0/jqc-1.12.4/dt-1.13.1/datatables.min.js"></script>
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/twitter-bootstrap/4.6.0/js/bootstrap.min.js"></script>
Keep note of the order - we have the bootstrap.min.css
first and then the datatables.min.css
. As for JavaScript, bootstrap.min.js
is after our DataTable javascript file
DataTables pagination styles with bootstrap 5 considerations
To use Boostrap 5 with Datatables we need to have the following loaded:
<link rel="stylesheet" type="text/css" href="https://cdnjs.cloudflare.com/ajax/libs/twitter-bootstrap/5.1.3/css/bootstrap.min.css"/>
<link rel="stylesheet" type="text/css" href="https://cdn.datatables.net/v/bs5/jqc-1.12.4/dt-1.13.1/datatables.min.css"/>
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/twitter-bootstrap/5.1.3/js/bootstrap.bundle.min.js"></script>
<script type="text/javascript" src="https://cdn.datatables.net/v/bs5/jqc-1.12.4/dt-1.13.1/datatables.min.js"></script>
Additionally, since Bootstrap 5 was a massive change over Bootstrap 4 and contains breaking changes we need to keep in mind of any CSS classes that we have used previously.
(An example of this massive change is dropping support of jQuery to use vanilla JS. This becomes a problem since DataTables is essentially a jquery plugin)
Some examples of breaking changes that could affect your table styings:
-
Breaking .thead-light and .thead-dark are dropped in favor of the .table-* variant classes which can be used for all table elements (thead, tbody, tfoot, tr, th and td). Examples of this are the
.table-primary
,.table-secondary
,.table-striped
, etc -
Renaming of -left and -right to -start and -end respectively. As an example
.text-left
and.text-right
becomes.text-start
and.text-end
.
Check out more of the breaking changes here:
Summary
In this post, I went over a few reasons why the pagination styles of DataTables is not loading correctly. It comes down to multiple reasons, but the main cause is that we are not loading the scripts and stylesheets correctly or in the wrong order.
Firstly, we need to make sure that jQuery is loaded before everything else. That is - jquery library comes first then the CSS and JS of the DataTables.
Another issue is due to not targetting the correct pagination classes that DataTable provides. We need to make sure that we are targeting the .paginate_button
class.
Finally, when using DataTables with Bootstrap, we need to carefully consider the version of Bootstrap we are using. Bootstrap 5 overhauls alot and got breaking changes over version 4. Additionally Bootstrap 5 drops support for jquery and instead uses vanilla JS.
So if we need support for browers such as IE9 then consider sticking with Bootstrap 4 version of DataTables!